Jobs in Sitecore
Sitecore Jobs are used to calling the task or method which takes more time to execute. It allows us to start and execute the task in the background without affecting the other task and thread's executions. We can monitor the jobs via Admin section in {Sitecore- Instance}/sitecore/admin/jobs.aspx page. So, in one line you can say Sitecore Jobs are perfect options to run the long execution methods.
Now the question is why Sitecore Job?
- Sitecore calls the <job> Pipeline to execute each job.
- Sitecore calls a separate thread for each job execution so that your code will be executed in the background without affecting any other method, thread block, etc.
- Sitecore also provided an admin section page to monitor the jobs.
- You can set the status and priority of the jobs.
- You can run multiple jobs at the same time without any interference.
- Sitecore Job provides job-related events like job:starting, job:started, job:ended, so that you can execute your custom code on these handlers.
Let have a quick example of a job, how can you start your job:
var jobAction = new Action(() => { var manager = ServiceLocator.ServiceProvider.GetService<IDataImport>(); manager.Import(); }); var options = new DefaultJobOptions("JobName", "Category", "WebsiteName", jobAction.Target, jobAction.Method.Name, null); var job = new DefaultJob(options); JobManager.Start(job); if (job.Handle == null) { job.Status.State = JobState.Finished; Log.Error("Could not start sitecore job.", new Exception(), GetType()); }
To define any job, first, you need to initialize the instance of the DefaultJobOptions class. It represents the options of the job and instructs Sitecore about what to do. Its constructor takes the below parameters:
- jobName: it needs to identify the job. Monitor page you can also use the same name to identify the job is running in the background or not and get the details of the job via code.
- category: Category name is used to groups the same type of jobs.
- siteName: Name of the website.
- Obj: It’s an object which contains a method that will execute. Here in the above example, I have created an object and named it jobAction. In this object I have created the instance of a method service class by dependency Injection and defined the method name as well that is Import().
- methodName: It’s the name of the method which we defined into the Obj parameter. Here I am using Method.Name to get the name of the method.
- object[] parameters: Parameters passed to run the method.
Next, you need to create the instance of the DefaultJob Class that represents the job and take JobOptions as a parameter.
After that, you need to create one more class instance that is JobManager. It represents the Job Manager. It provides a method named Start() to trigger the job. Its also provides some other method like:
- GetJobs(string jobName): Get the Job by job Name.
- IsJobQueued(string jobName): determine whether the specified job name in the parameter is queued or not.
- IsJobRunning(string jobName): Determine whether the specified job name in the parameter is running or not.
- Start(BaseJob job): start the specified job.
So, you can say Sitecore Job use, three classes, to work with the jobs:
- Sitecore.Jobs.DefaultJobOptions
- Sitecore.Jobs.DefaultJob
- Sitecore.Jobs.JobManager
Job Status: There is one more class named Sitecore.Jobs.JobState.It's an enum class that indicates the job status(Initializing, Queued, Running, Finished, Unknown). You can set the status of the job like:
job.Status.State = JobState.Finished;
Priority: You can set the priority of the job. It is possible by thread priority.
var options = new DefaultJobOptions({your parameters}) options.Priority = System.Threading.ThreadPriority.Lowest; JobManager.Start(options);
Priority can be Lowest, BelowNormal, Normal, AboveNormal, and Highest.
Events: Jobs provide three written events:
- OnJobStarting: Raised before a job is placed into the thread pool
- OnJobStarted: Raised after a job is placed into the thread pool and has been started
- OnJobEnded: Raised during the Job_Finished event
For example:
public virtual void OnJobStarting(Object sender, EventArgs e) { if (e is SitecoreEventArgs) { //your code } }
Monitor Sitecore Jobs: To check Sitecore jobs running in the background, login to the Sitecore interface and go to the admin page that is: {Sitecore-isntance}/sitecore/admin/jobs.aspx.
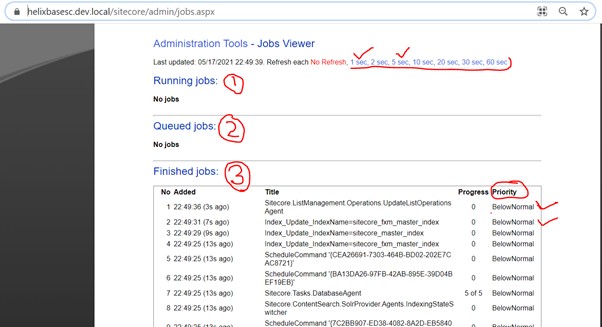
It has three sections:
- Running Jobs: In this section background running jobs will be displayed.
- Queued Jobs: In this job displayed will be in the queue for execution.
- Finished Jobs: List of the completed jobs.
In the above image, you can identify that all the listed jobs in the Finished Jobs section priority is set to the BelowNormal. You can select the page refresh option from the top section to refresh the jobs viewer.
Happy Sitecoreing 😊
Comments
Post a Comment